Blog
All the latest info and insights from the Akka team
The latest
Lightbend is now Akka
Today, we are announcing: Akka 3 - the next gen of Akka, our product unification into Akka, a new Akka.io, and Lightbend is now doing business as Akka!
Multi-region replicated apps
With Akka, create multi-region stateful applications that ensure low latency, no-downtime disaster recovery, seamless upgrades, and effortless cross-cloud migrations.
All posts
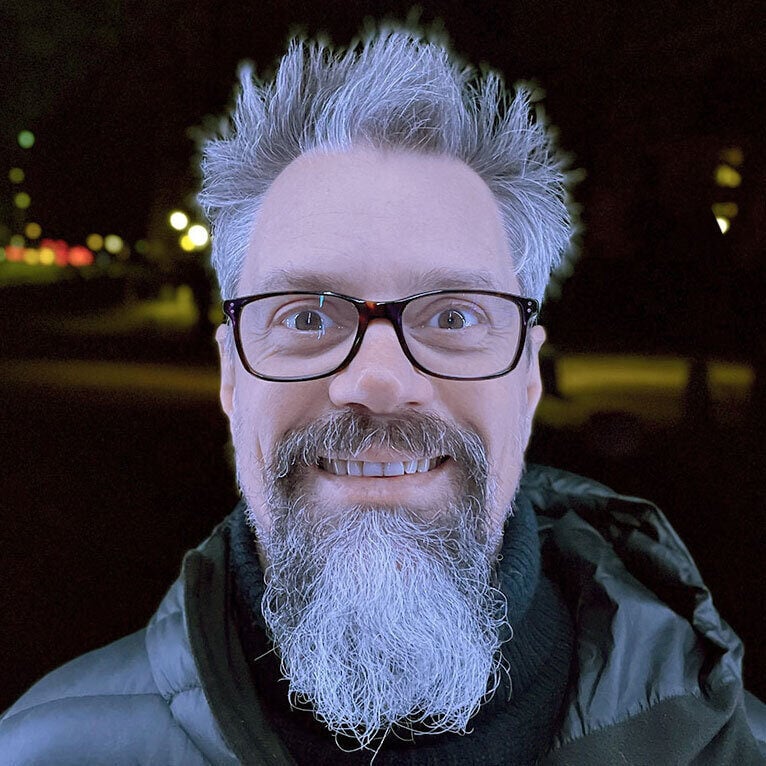
Jonas Bonér
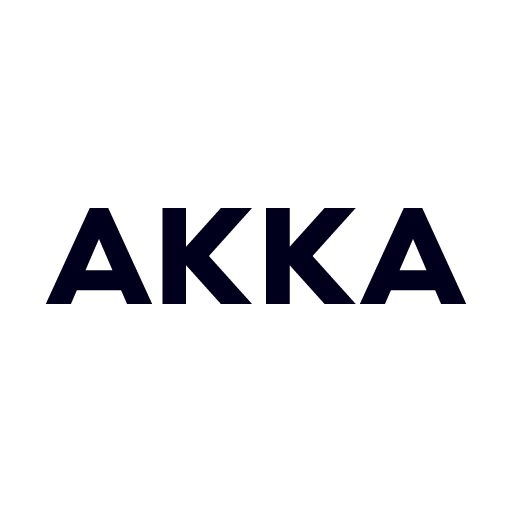
Team Akka
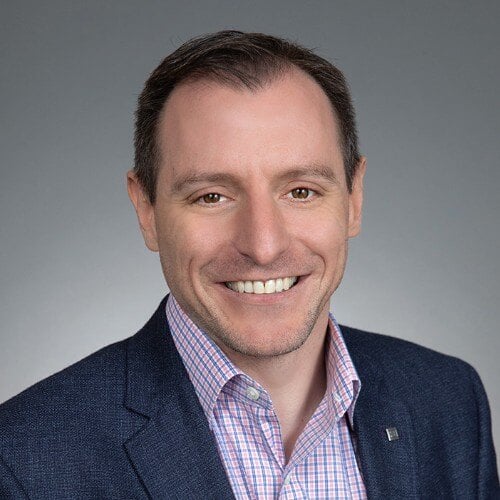
Tyler Jewell
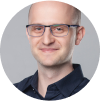
Andrzej Ludwikowski
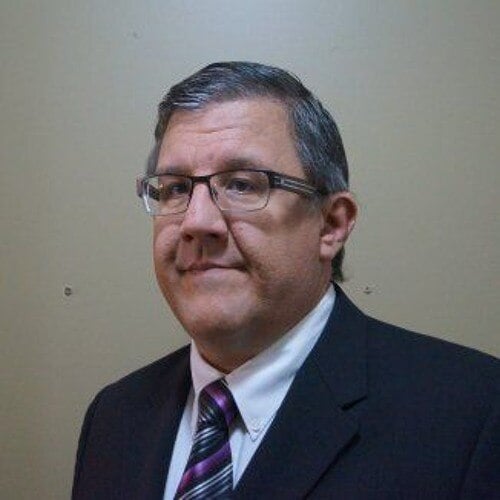
Michael Nash
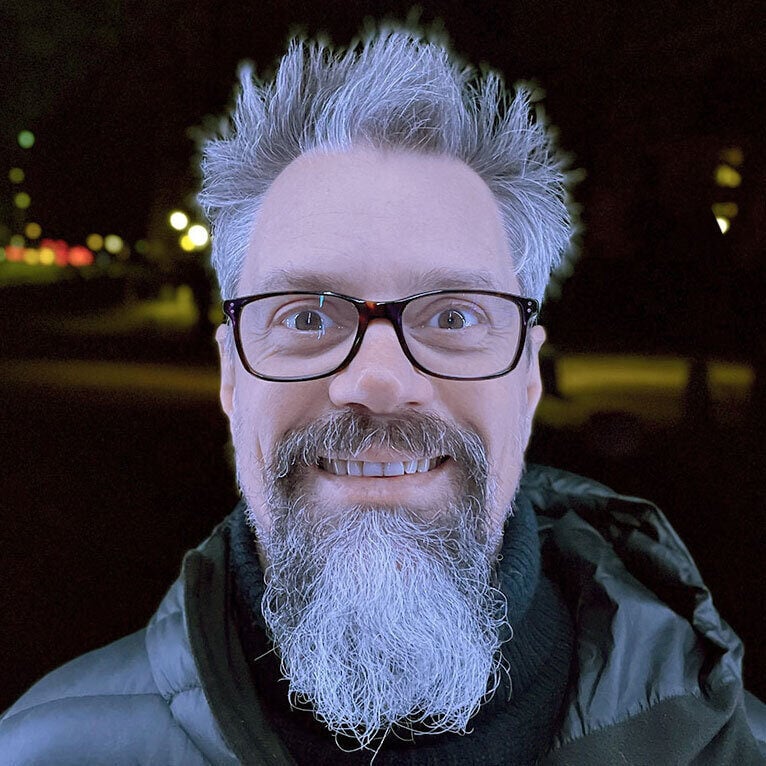
Jonas Bonér
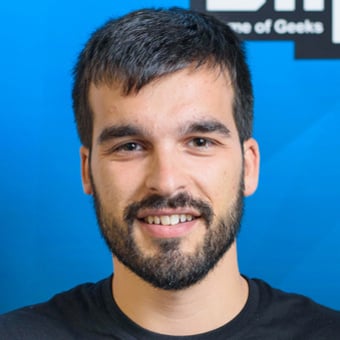
Eduardo Pinto
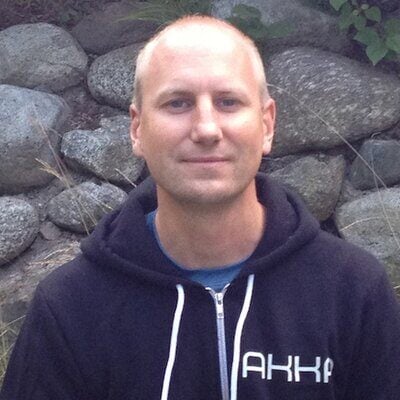
Patrik Nordwall
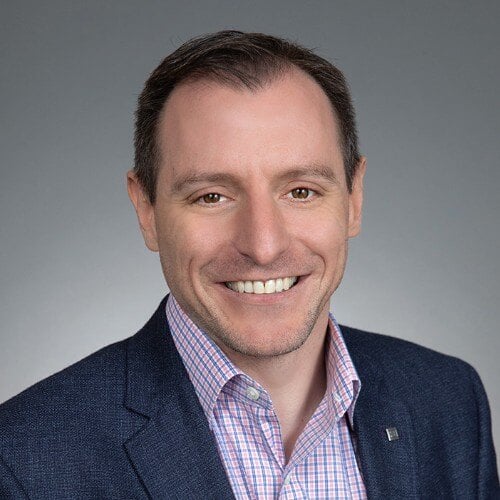
Tyler Jewell
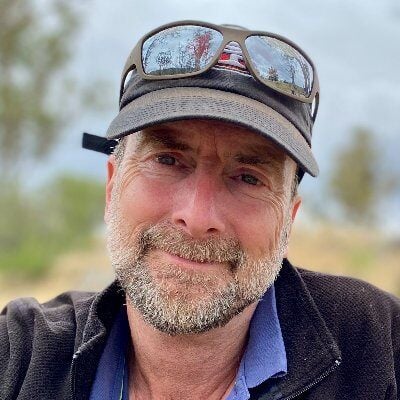
Christopher Hunt
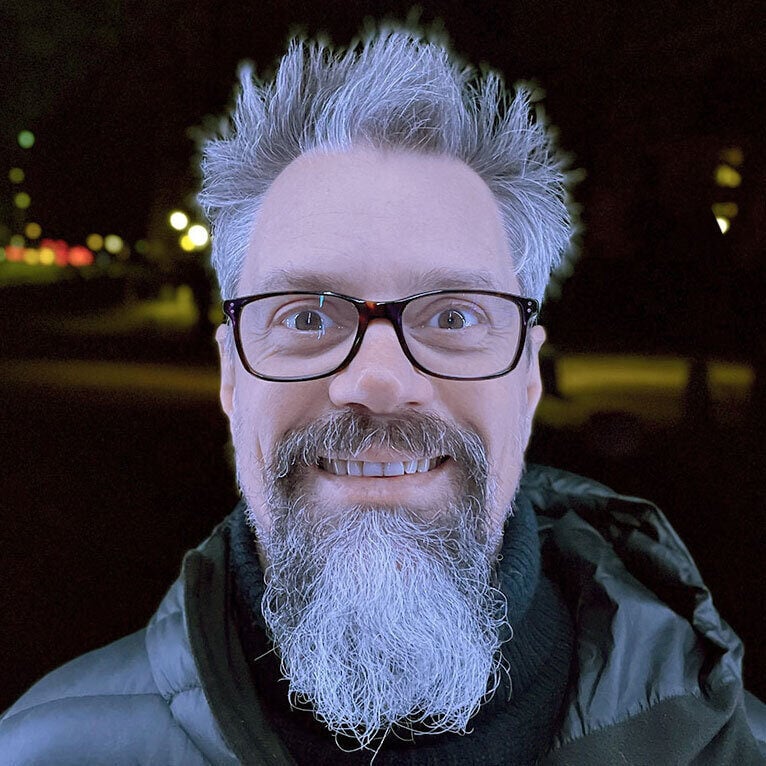
Jonas Bonér
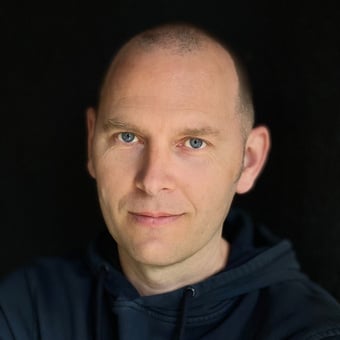
Peter Vlugter
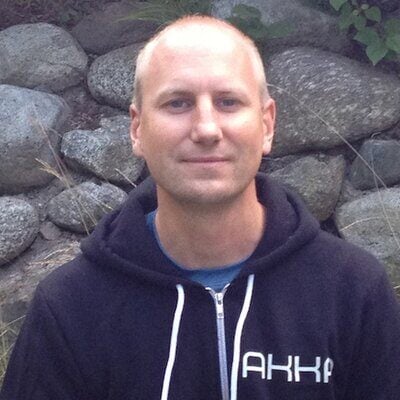
Patrik Nordwall
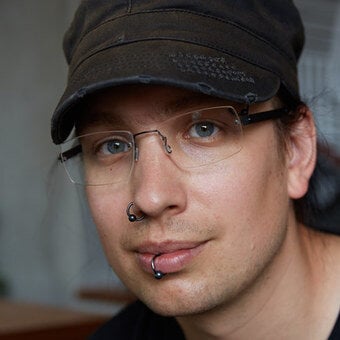
Johan Andrén
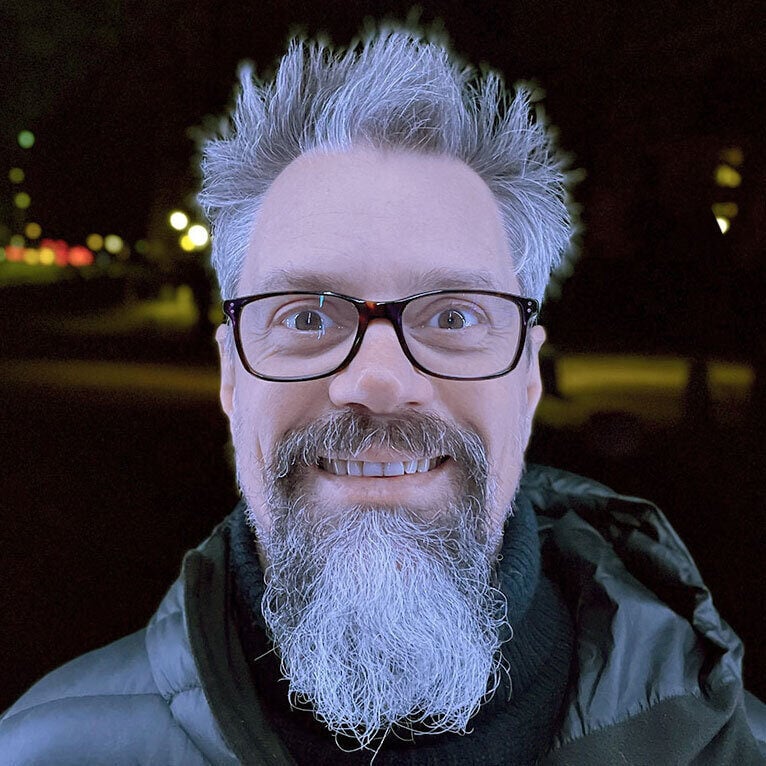
Jonas Bonér
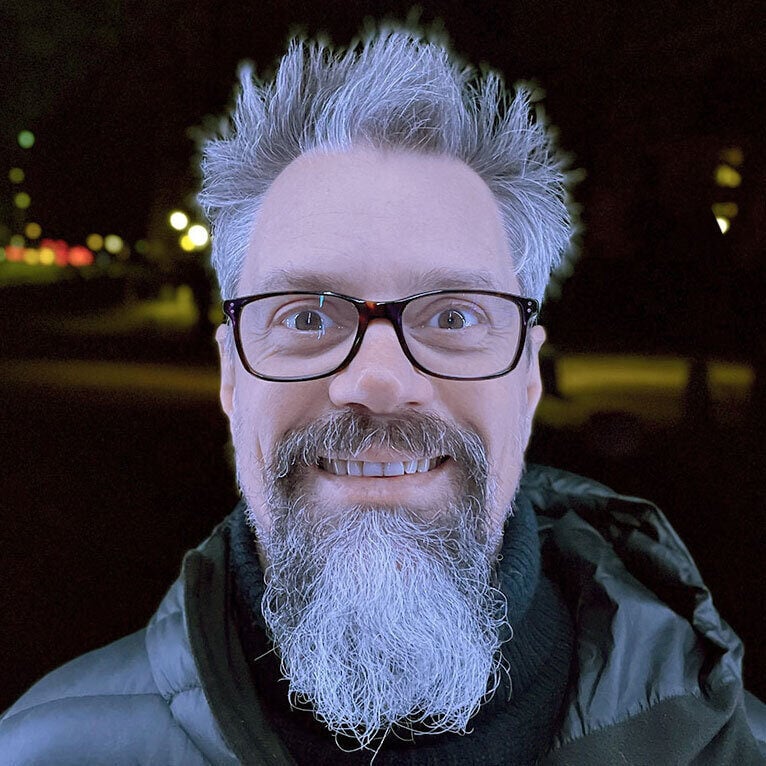
Jonas Bonér
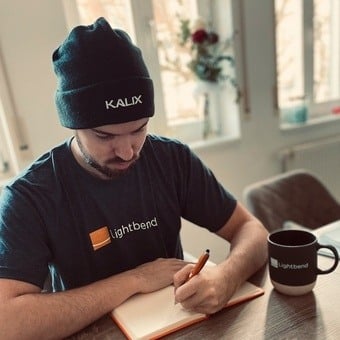
Janik Dotzel
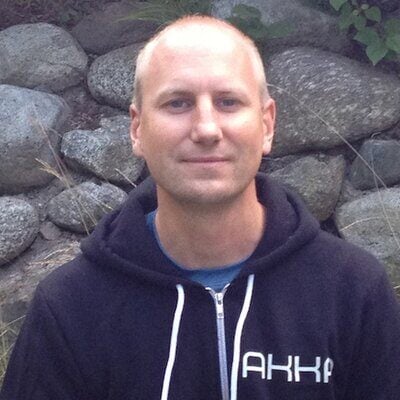
Patrik Nordwall
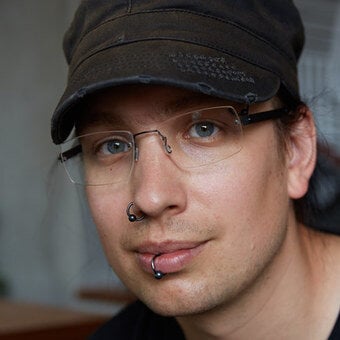
Johan Andrén
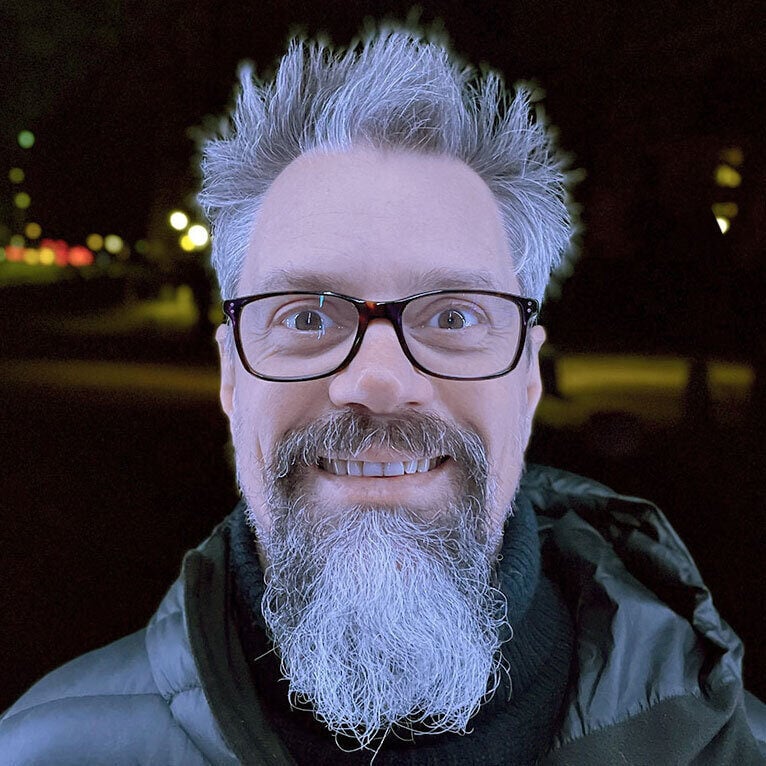
Jonas Bonér
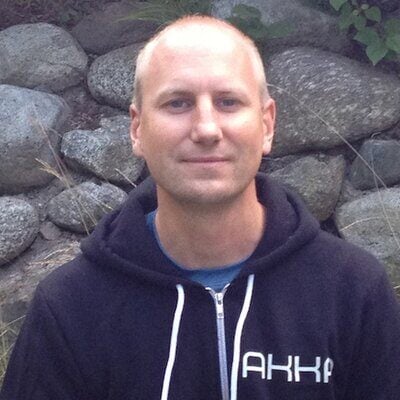
Patrik Nordwall
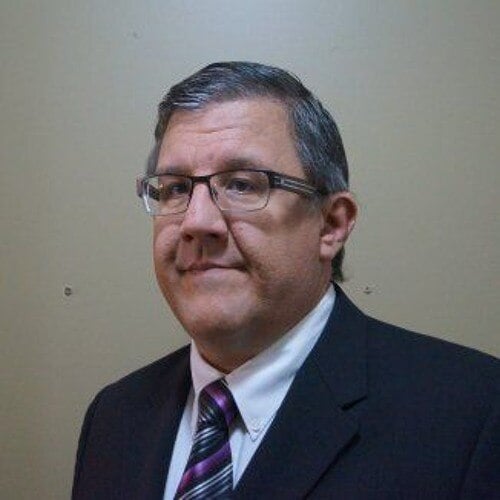
Michael Nash
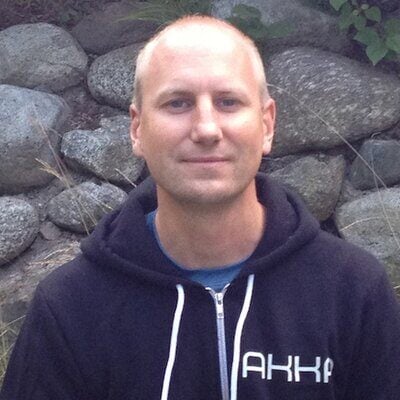
Patrik Nordwall
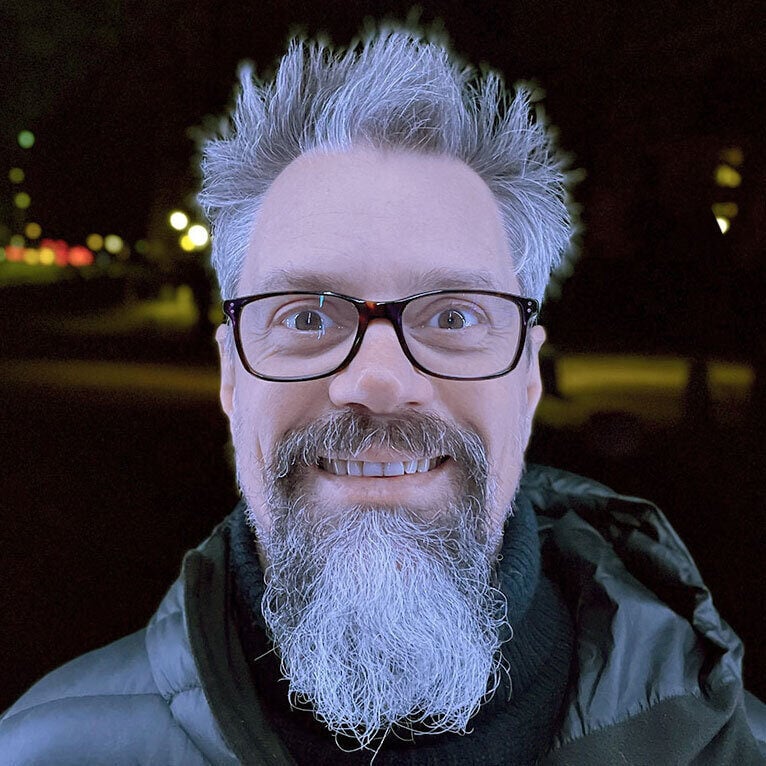
Jonas Bonér
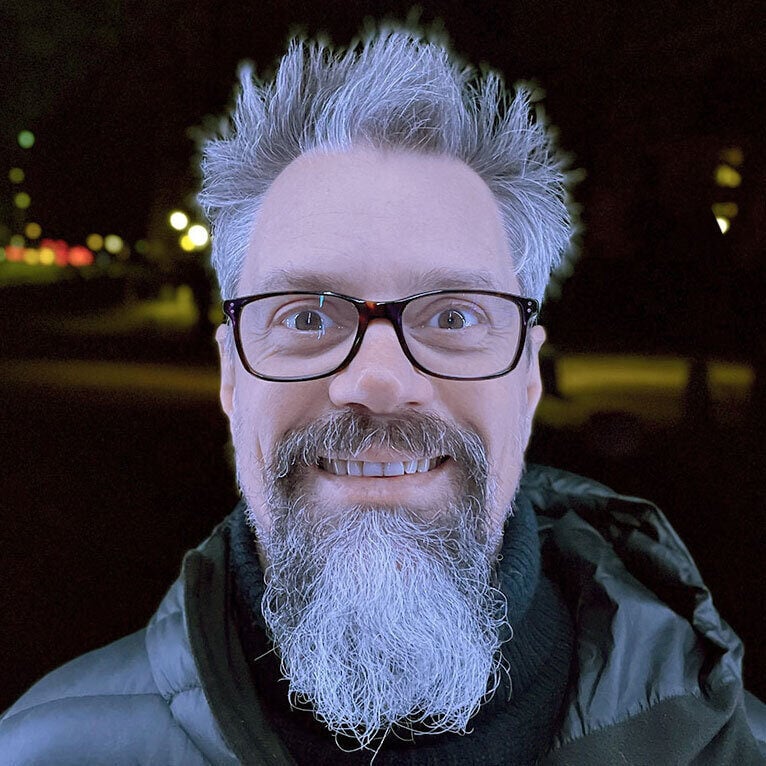
Jonas Bonér